What is a container?
A container is a standard unit of software that packages up code and all its dependencies so that the application can run quickly from one computing environment to other.
Host App/Website On Docker Container
First, We have to create a Dockerfile for building an image for our website hosting.
FROM ubuntu:20.04
ENV TZ=Asia/KolkataMAINTAINER Sarthak-Dixit
RUN apt-get update -y \
&& apt-get install tzdata \
&& apt-get install -y apache2 wget curl \
&& apt-get install -y unzip sed\
&& a2enmod rewrite \
&& export LANG=en_US.UTF-8 \
&& apt-get install -y software-properties-common \
&& apt-get install -y language-pack-en-base \
&& add-apt-repository ppa:ondrej/php -y\
&& apt-get update -y \
&& apt-get install -y php8.1 php8.1-mysql php8.1-bcmath php8.1-gd php8.1-bz2 php8.1-xml php8.1-cli php8.1-common php8.1-intl php8.1-curl php8.1-zip php8.1-mbstring php8.1-soap libapache2-mod-php8.1 \
&& rm /var/www/html/* \
&& wget https://wordpress.org/latest.zip \
&& unzip latest.zip \
&& mv wordpress/* /var/www/html \
&& cd /var/www/html \
&& cp wp-config-sample.php wp-config.php \
&& sed -i -e ‘s/database_name_here/wordpress/g’ /var/www/html/wp-config.php \
&& sed -i -e ‘s/username_here/root/g’ /var/www/html/wp-config.php \
&& sed -i -e ‘s/password_here/qwerty/g’ /var/www/html/wp-config.php \
&& sed -i -e ‘s/localhost/mysql/g’ /var/www/html/wp-config.phpEXPOSE 80
RUN apt-get install -y supervisor \
&& mkdir -p /var/log/supervisor
env APACHE_RUN_USER www-data
env APACHE_RUN_GROUP www-data
env APACHE_PID_FILE /var/run/apache2.pid
env APACHE_RUN_DIR /var/run/apache2
env APACHE_LOCK_DIR /var/lock/apache2
env APACHE_LOG_DIR /var/log/apache2COPY supervisord.conf /etc/supervisor/conf.d/
WORKDIR /var/www/html
CMD [“/usr/bin/supervisord”]
Copy the code and save it as file-name of Dockerfile with your file editor.
And secondly, create a supervisor configuration file.
[supervisord]
nodaemon=true[program:apache2]
command=/bin/bash -c “source /etc/apache2/envvars && exec /usr/sbin/apache2 -DFOREGROUND”
Copy the code and save it as file-name of supervisord.conf with your file editor.
Note: The file “supervisord.conf” is required to start the web-server service in the container and we can use this file to start other services as well.
Now, We are building an image by using the above-created Dockerfile.
$ docker build -t wp-image .
The above command builds an image with the name wp-image. You can list all your docker images by using the command “docker images”.
After building the image, We have to run the build image on the container.
Firstly, We run a MySQL container to store the database.
$ docker run -d –name mysql-cont -e MYSQL_ROOT_PASSWORD=qwerty -e MYSQL_DATABASE=wordpress mysql:5.7
And in the above command, We run the container with a detached terminal by “-d”, name of “mysql-cont” by using “–name”, Create a database by using “ -e MYSQL_DATABASE=wordpress“, Configure password in mysql root user by “-e MYSQL_ROOT_PASSWORD=” and using mysql:5.7 image in which “mysql” is image name and “:5.7” is version tag.
Then, We run a container of our build image.
$ docker run -dit –name wordpress –link mysql-cont:mysql -p 8080:80 wp-image
In this command, Everything is the same as have we run the previous command of MySQL container except “–link”, “-it” and “-p”.Here, “-it” means instruct docker to give terminal interaction to the container.
For creating links between containers, We use “–link” to link the WordPress container to the MySQL container for sharing resources of the database container.
In porting while running a container we use “-p” to attach the external port to the container’s port.
8080 — refers base machine’s port.
80 — refers container’s port.
After all the above processes, you will be able to browse the website at http://localhost:8080.
Dockerfile
Dockerfile is a text file that includes all the commands a user can call on the command line to bring together an image.
Commands Use In Dockerfile Environment
FROM — Uses for fetching and using images from the base machine if present, otherwise it will get it from the docker registry.
RUN — For running commands in shell form over the image.
CMD — Use to execute the command and It will be written in an executable set as [parameter-1, parameter-2].
MAINTAINER — Use for maintaining Dockerfile author.
EXPOSE — For exposing the port of the image.
WORKDIR — For setting the working directory of the image.
ADD — Use for copying our local machine data to docker image as well as use URL to copy and extract tar directory from source to destination.
COPY — For copying our local machine’s data to the docker image.
env — For setting the environment in the docker image.
IP Allocation To Container/Assign Static IP To Container
First, we have to create a network on docker.
$ docker network create –subnet=10.6.0.0/16 mynetwork
And then, we run our container by using this network and assigning the static IP.
$ docker -d run –net mynetwork –ip 10.6.0.1 -p 8080:80 –name server wp-image
Communication between docker containers
A bridge network is an easy and default way to communicate between containers. Containers which is under the same bridge network will able to communicate by name or alias.
$ docker network ls
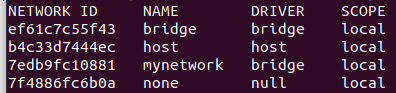
By using the above command, you will able to list the docker network which includes Network ID, Network Name, Driver, and Scope.
Note: The container’s communication is based on IP within the same network of the bridge and also by name or alias of the container.
This is the default way to communicate or connect between containers within the default bridge.
User Defined Docker Network
$ docker network create Bnet
$ docker run –net Bnet -p 80:8080 –name server ubuntu/apache2
$ docker run –net Bnet -p 8080:8080 –name ubuntu-server wp-image
In the first command, We are creating a network in the docker network as default with a bridge network using the name “Bnet”.
In the second and third commands, We are creating a container under the “Bnet” network.
The container of php:8.0-apache and wp-image will communicate because of containers within a user-defined bridge network.
Docker Volume
Let’s create a docker volume for persisting container data. So, We are going to create a volume to save MySQL data.
$ docker volume create mysql-data
And we are going to use created volume to save data while starting a container of the “mysql:5.7” image.
$ docker run -d –name mysql-cont -v mysql-data:/var/lib/mysql -e MYSQL_ROOT_PASSWORD=qwerty mysql:5.7
After using persisting volume “-v mysql-data:/var/lib/mysql“, the Data of MySQL will save in “mysql-data” docker volume.
If you are not using attribute “-v” to create a docker volume, Docker will create a volume for your container.
Mount Directory To Container
By default docker will create volume when you run the container by using an attribute with “-v xyz:/path”. Here “xyz” is the volume of the docker created during the docker run with “-v” attribute.
If you use “-v /path-of-local-directory:/path-of-container-directory” then it mounts and persists data on the base machine’s directory to the container’s directory.
You can display a list of docker volumes by the following command :
$ docker volume ls
If you want docker volume details then you will display them by inspecting that volume using the following command :
$ docker volume inspect <volume-name>
Why do we need persistent volume?
We need persistent volume to protect the data loss if the containers get destroyed or crash. Therefore, the data will remain safe in the base machine even if the container gets destroyed because of persisting volume and mount directory.
For Magento 2 Elasticsearch, please follow the –
Our Cloudkul Blogs
Elasticsearch, Fluentd, and Kibana (EFK)
Setting up Elasticsearch, Logstash, and Kibana for centralized logging
Managing and Monitoring Magento 2 logs with Kibana
Our store modules –
You may also visit our Magento development services and quality Magento 2 Extensions.
For further help or query, please contact us or raise a ticket.